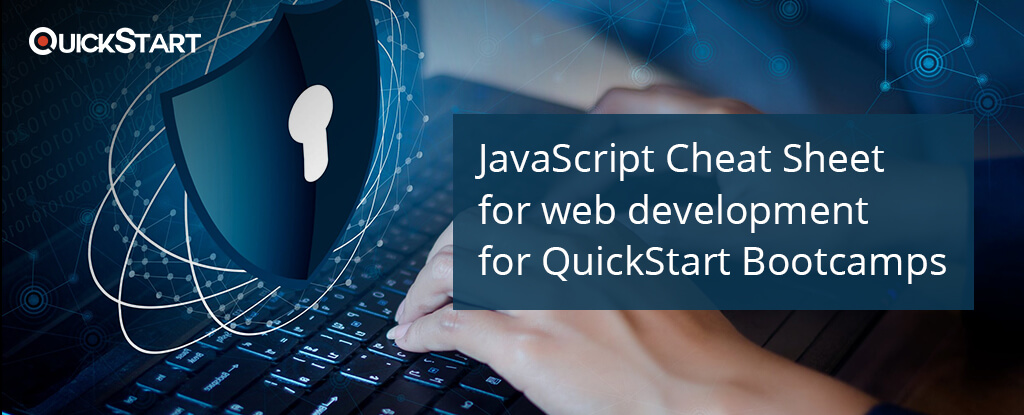
JavaScript Cheat Sheet for Web Development?
JavaScript is a programming language mostly used by web developers to create web pages and applications to provide an interactive and dynamic user experience. Most of the web-based applications and functions that make the Internet vital to modern-day life are coded in JavaScript in form or another.
JavaScript is an old language as it started in the late 1990s. The fact that JavaScript is currently used by 94.5% of all websites highlights the importance of JavaScript as a programming language. Being a client-side programming language, JavaScript helps web developers to create websites dynamic and interactive by implementing the custom scripts.
Simultaneously, the web developers also use cross-platform runtime software like Node.js to write server-side codes in JavaScript. Web developers can even put up a combination of JavaScript, HTML5, and CSS3 to create web pages that work across various browsers, platforms, and devices. There are also several other reasons which make JavaScript a must-have tool under the belt for modern-day web developers to get all benefits of it.
This article is intended to work as a Web Development boot camp to understand and learn JavaScript. We will go through the JavaScript cheat sheet to facilitate young aspirants of web development.
Enroll in our Web Development bootcamp today
JavaScript Cheat Sheet
Basics
Including JavaScript in HTML Page
To include JavaScript inside a web page, wrap it in <script> tags
<script type="text/javascript">
//Add JS code here
</script> |
Including External JavaScript File
JavaScript can also be placed in its own file and be named it inside HTML
<script src="filename.js"></script> |
Adding Comments
Comments let other people understand what is going on in your code or remind you if you forget something.
One line comments: // Multi-line comments: /* comment here */ |
Variables
Variables are those values that you can use to perform operations
var, const, let
There are three different possibilities for using a variable in JavaScript with their unique specialties:
var: The most common variable. Can be reallocated but only accessible within a function. Const: Can’t be reassigned and not accessible before appearing within the code. let: Just like const, however, let variable can be reassigned |
Data Types
Variables contain different types of values and data types which are assigned by using =
Numbers: var age = 25 Variables: var x Text (strings): var a = "init" Operations: var b = 1 + 2 + 3 True or False statements: var c = true Constant numbers: const PI = 3.14 Objects: var name = {firstName:"John", lastName:”Wick"} |
Objects
These are certain kinds of variables with their own values and methods
var student = { firstName:"John", lastName:"Wick", age:25, nationality:"British" }; |
Arrays
Arrays are part of several programming languages. They are used to organize variables and properties into groups
var dogs = ["Poodle", " Bulldog ", "Labrador"]; |
Array Methods
Once arrays have been created, there are a few things you can experiment with them
concat( ): Join various arrays into one indexOf( ): Returns the first position of given element at which it appears in an array join( ): Combine elements of an array into a single string and return it lastIndexOf( ): Gives the last position of given element at which it appears in an array pop( ): Eliminates the last element of an array push( ): Add new element at the end reverse( ): Sort elements in a descending order shift( ): Remove the first element of an array slice( ): Pulls a copy of a portion of an array into a new array sort( ): Sorts elements alphabetically splice( ): Adds elements in a specified way and position toString( ): Converts elements to strings unshift( ): Adds a new element to the beginning valueOf( ): Returns the primitive value of the specified object |
Enroll in our Web Development bootcamp today
Operators
Operators are used for performing different operations with variables
Basic Operators
+ : Addition - : Subtraction *: Multiplication / : Division (...) : Grouping. Operations within brackets are executed first %: Modulo (remainder ) ++ : Postfix Increment -- : Postfix Decrement |
Comparison Operators
== : Equal to === : Both equal value and type != : Not equal !== : Neither equal value nor equal type > , < : Greater than, Less than >= : Greater than or equal to <= : Less than or equal to ?: Ternary operator |
Logical Operators
&& : Logical AND || : Logical OR !: Logical NOT |
Bitwise Operators
& : AND | : OR ~ : NOT ^ : XOR << : Left Shift >> : Right Shift >>> : Zero Fill Right Shift |
Functions
In JavaScript functions are blocks of code to perform a certain task. A basic function may look like as under:
function name(parameter1, parameter2, parameter3) { // function role } |
The function’s parameters are given in the brackets. Moreover, the task of a function is written in curly brackets.
Output of Data
Functions are commonly used for the output of data. For the output, the following options are available:
alert() : Display data in an alert box confirm() : Opens up a yes/no dialogue box and returns true/false depending on user response console.log() : Transcribes information to the browser console document.write() : Transcribes directly to the HTML document prompt() : User input dialogue box |
Global Functions
Global functions are universal functions which are built into every browser capable of running JavaScript:
decodeURI() : Decodes a Uniform Resource Identifier (URI) produced by encodeURI decodeURIComponent() : Decodes a URI component encodeURI() : Encodes URI into UTF-8 encodeURIComponent() : Same as above but for URI components eval() : Evaluates JS code presented as a string isFinite() : Defines if a passed value is a finite number isNaN() : Defines whether a value is NaN or otherwise Number() : Returns a number transformed from its argument parseFloat() : Analyzes an argument and returns a floating-point number parseInt() : Analyzes its argument and returns an integer |
JS Loops
Loops are included in most programming languages. They allow developers to execute blocks of code several times with different values:
for (before loop; condition for loop; execute after loop) { // what to do within the loop } |
Some parameters to create loops are:
for : The most used way to create a loop in JS while : Lay down conditions under which a loop executes do while : Similar to the while loop but it executes at least once break : Used to stop and exit the cycle at specific conditions continue : Skip parts of the cycle if specific conditions are fulfilled |
If – Else Statements
Easily understandable, using these statements, you can set conditions for when your code is executed
if (condition) { // what to do if condition is fulfilled } else { // what to do if condition is not fulfilled } |
Strings
Strings in JS are the text that does not perform any function but can appear on the screen
var person = "John Wick"; |
Escape Characters
In JS, if you want to use quotation marks within a string, you need to use special characters
\' : Single quote \" : Double quote \\ : Backslash \b : Backspace \f : Form feed \n : New line \r : Carriage return \t : Horizontal tabulator \v : Vertical tabulator |
String Methods
There are several ways to use strings
\charAt() : Returns a character to a specified position charCodeAt() : Provides the Unicode of a character at that position concat() : Concatenates (connects) two or more strings fromCharCode() : Returns a string created from the specified sequence of UTF-16 code indexOf() : Provides the position of the first incidence of a specified text within a string lastIndexOf() : Same as above but with the last occurrence match() : Retrieves the matches of a string against a search pattern replace() : Find and replace specific text in a string search() : Executes a search for a matching text slice() : Extracts a section of a string as a new string split() : Splits a string object into an collection of strings substr() : Similar to slice() but extracts a substring substring() : Like slice() but can’t accept negative guides toLowerCase() : Change strings to lower case toUpperCase() : Change strings to upper case valueOf() : Returns the original value of a string object |
Regular Expression Syntax
These are search patterns used to match character combinations in strings
Pattern Modifiers
e : Evaluates a replacement i : Carry out case-insensitive matching g : Carry out global matching m : Performs multiple line matching s : Treats strings as a single line x : Allow comments in the pattern |
Meta-characters
. : Find a single character \w : Word character \W : Non-word character \d : A digit \D : A non-digit character \s : Whitespace character \S : Non-whitespace character \b : A match at the beginning or end of a word \B : A match not at the beginning or end of a word \0 : NUL character \n : A new line character \f : Form feed character \r : Carriage return character \t : Tab character \v : Vertical tab character \xxx : The character specified by an octal number xxx \xdd : Character specified by a hexadecimal number dd \uxxxx : Unicode character specified by a hexadecimal number XXXX |
Quantifiers
n+ : Matches any string with at least one n n* : String with zero or more occurrences of n n? : String with zero or one occurrence of n n{X} : String with a sequence of X n’s n{X,Y} : Strings that contain a sequence of X to Y n’s n{X,} : Matches any string with a sequence of at least X n’s n$ : String with n at the end of it ^n : String with n at the beginning of it ?=n : Any string that is followed by a specific string n ?!n : String that is not followed by a specific string ni |
Start your 7-day FREE TRIAL with QuickStart to learn web development and choose from self paced courses or virtual instructor led classes.
Numbers and Math
In JS, you can perform mathematical functions.
Number Properties
MAX_VALUE : Maximum numeric value MIN_VALUE : Smallest positive numeric value NaN : “Not-a-Number” value NEGATIVE_INFINITY : Negative Infinity value POSITIVE_INFINITY : Positive Infinity value |
Number Methods
toExponential() : Returns the string with a rounded number toFixed() : Returns the string with a specified number of decimals toPrecision() : String of a number written with a specified length toString() : Returns a number as a string valueOf() : Returns a number as a number |
Math Properties
E : Euler’s number LN2 : Natural logarithm of 2 LN10 : Natural logarithm of 10 LOG2E : Base 2 logarithm of E LOG10E : Base 10 logarithm of E PI : The number PI SQRT1_2 : Square root of 1/2 SQRT2 : The square root of 2 |
Math Methods
abs(x) : Returns the positive value of x acos(x) : The arccosine of x asin(x) : Arcsine of x atan(x) : Arctangent of x as a numeric value ceil(x) : Value of x rounded up to its nearest integer cos(x) : The cosine of x in radians exp(x) : Value of Ex floor(x) : The value of x rounded down to its nearest integer pow(x,y) : X to the power of Y sin(x) : The sine of x (x is in radians) tan(x) : Tangent of an angle |
Dates in JS
JS also allows people to work with date and time
Setting Date
Date() : Creates a date object with the current date and time Date(2020, 7, 13, 2, 45, 10, 0) : Custom date object with a year, month, day, hour, minutes, seconds, milliseconds Date("2020-07-13") : Date as a string |
Date and Time Values
getDate() : Day of the month (1-31) getDay() : Weekday as a number (0-6) getFullYear() : Year (yyyy) getHours() : Hour (0-23) getMinutes() : Minute (0-59) getMonth() : Month as a number (0-11) getUTCDate() : The day (date) of the month according to universal time |
User Browser
JS is also enabled to take into account the user browser and incorporate the properties in the code.
Window Properties
closed : Whether a window has been closed or not defaultStatus : Sets or returns the default text in the status frames : All <iframe> elements in the current window history : Provides the History object for the window innerHeight : Inner height of content area innerWidth : Inner width of content area length : Number of <iframe> elements in the window location : Returns the location object for the window name : Sets or returns the name of a window navigator : Returns the Navigator object for the window outerHeight : Outer height including toolbars/scrollbars outerWidth : Outer width including toolbars/scrollbars pageXOffset : Number of pixels horizontally pageYOffset : Number of pixels vertically parent : Parent window of the current window screenLeft : Horizontal coordinate of the window screenTop : Vertical coordinate of the window self : Returns the current window |
Window Methods
alert() : Displays an alert box close() : Closes the current window confirm() : Dialogue box with an OK and Cancel button open() : Opens new browser window print() : Prints the content prompt() : Dialogue box for visitor’s input stop() : Stops the window from loading |
JS Events
In JS, Events are things which can happen to HTML elements as performed by the user.
Mouse
onclick :When the user clicks on an element oncontextmenu : User right-clicks on an element ondblclick : Double click on an element onmousedown : User presses a mouse button over an element onmouseenter : Pointer moves onto an element onmouseleave : Pointer moves away from an element onmouseup : User releases a mouse button while over an element |
Keyboard
onkeydown : User pressing a key down onkeypress : When user starts pressing a key onkeyup : User releases a key |
Frame
onabort : Loading of a media is terminated onbeforeunload : It occurs before the document is about to be unloaded onerror : Error while loading an external file onhashchange : Changes to the anchor part of a URL onload : Object has loaded onpagehide : User go away from a webpage onpageshow : User comes to a webpage onresize : Document view is resized onscroll : Element’s scrollbar is scrolled onunload : It occurs when a page has unloaded |
QuickStart's LITE Subscription costs zero dollars and provides access to IT courses, learning analytics to help you monitor your learning progress, access to expert community and informal learning resources. Start learning free of cost!
Form
onblur : An element loses focus onchange : The content of a form element changes onfocus : Element gets focus onfocusin : When an element is about to get focus onfocusout : Element is about to lose focus oninput : User input on an element oninvalid : Element is invalid onreset : A form is reset onsearch : User enters something in a search field onselect : Text selection by user onsubmit : Form is submitted |
Drag
ondrag : When element is dragged ondragend : User has completed dragging the element ondragenter : Dragged element gets a drop target ondragleave : Dragged element leaves the drop target ondragover : Dragged element comes on top of the drop target ondragstart : User starts to drag an element ondrop : Dragged element is dropped on the target |
Clipboard
oncopy : Content of an element copied by the user oncut : User cuts an element’s content onpaste : Content is pasted by the user |
Media
onabort : Loading is cancelled oncanplay : Media can start playing by browser ondurationchange : Duration of the media changes onerror : While loading an external file error occurs onloadeddata : Date of media is loaded onloadstart : Browser looks for specified media onpause : Media is paused onplay : The media is on play onprogress : Media is downloading by browser onseeking : User starts moving / skipping onsuspend : Browser is deliberately not loading media ontimeupdate : Media playing position has changed onvolumechange : Change in media volume (including mute) onwaiting : Media paused but likely to resume (e.g., buffering) |
Animation
animationend : CSS animation has been completed animationiteration : CSS animation has been repeated animationstart : CSS animation has started |
Others
transitionend : Dismissed on a CSS transition completion onmessage : Message is received via event source onoffline : Browser starts working offline ononline : Browser starts working online onpopstate : Window’s history changes onshow : A <menu> element is displayed as a context menu onstorage : Web Storage area has been updated ontoggle : The <details> element is opened or closed by user onwheel : Mouse wheel scrolls up or down above an element ontouchcancel : Screen-touch has been disturbed ontouchend : User’s finger is detached from a touch-screen ontouchmove : A finger has been dragged on the screen ontouchstart : Touch of finger is placed on the screen |
Errors
In JS, different errors can occur and there are several ways of coping with them
try : Allows you set up a block of code for testing errors catch : Define a block of code to execute in case of an error throw : Instead of the standard JS errors it creates custom error messages finally : Allows you execute code irrespective of the result, after try and catch method |
Errors Names & Values
JS also has a built-in error object with a couple of properties: name : Sets or yields the name of error message : Sets or returns an error message in a form of string The error property can result in six different values: EvalError : Error occurs in the eval() function RangeError : Number is “out of range” ReferenceError : An prohibited reference has happened SyntaxError : A syntax error has happened TypeError : A typo error has happened URIError : encodeURI() error has occurred |
The JavaScript Cheat Sheet – Final Word
JavaScript, since its inception, has been continuously gaining importance as a programming language. In the JavaScript cheat sheet compiled above, most of the basic and essential functions, operators, principles, and methods have been explained. It provides a holistic overview of the language and can be taken as a reference for developers and new learners.
Connect with our experts for guidance on upskilling or starting a career in web development.